A slow but token-efficient sort:
-- 35-token bubblesort, by pancelor function sort(arr) for i=1,#arr do for j=i,#arr do if arr[j] < arr[i] then add(arr,deli(arr,j),i) --slow swap end end end end |
I did a brief speed analysis and this function seems reasonable to use for arrays up to around 50 or 100 elements, depending on how much CPU you have available to burn.
speed analysis:


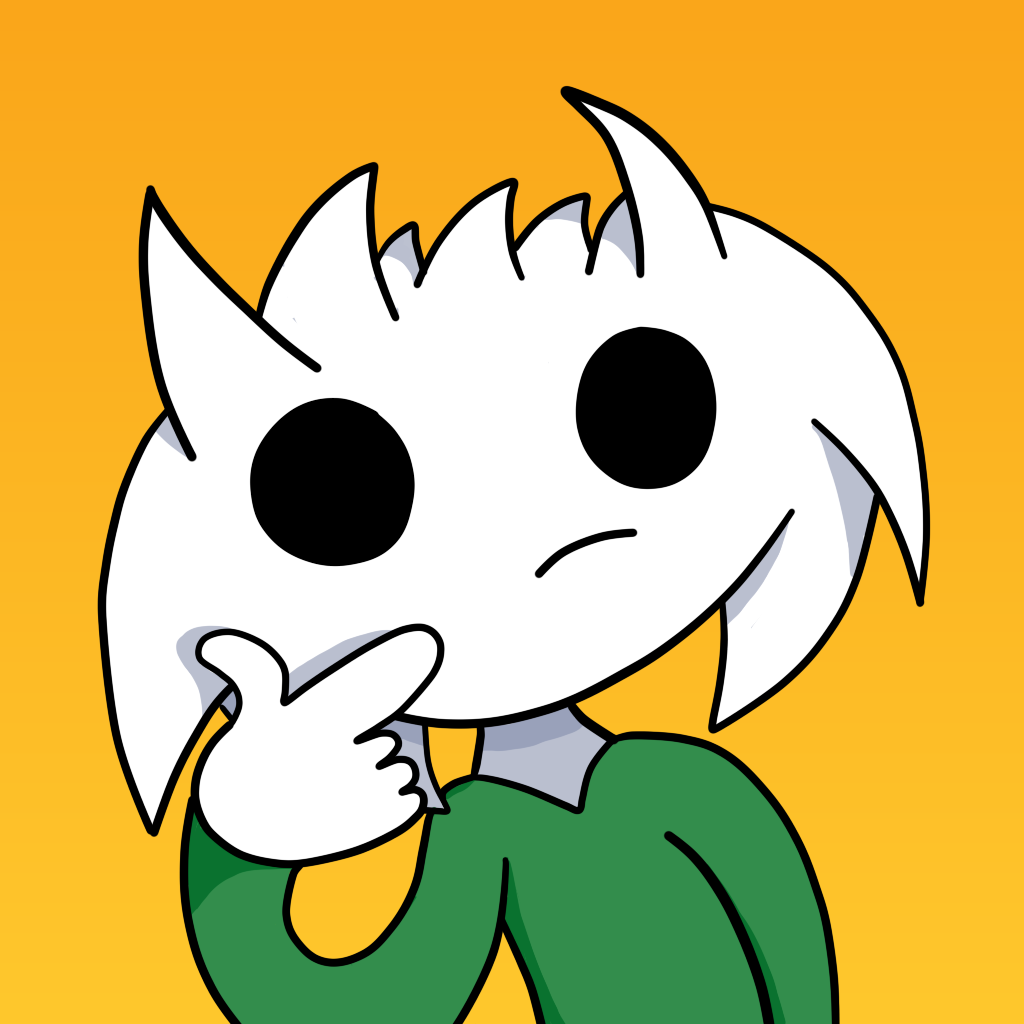
I found a sorting implementation that's 1 token cheaper (34 tokens), and slightly better cpu wise - based on this simple and surprising sorting algorithm: https://arxiv.org/pdf/2110.01111.pdf
function sort(arr) for i,arri in inext,arr do for j,arrj in inext, arr do if arri<arrj then arri,arr[i],arr[j]=arrj,arrj,arri end end end end |
complexity is clearly n^2, and also a bit better cpu wise on your tests (for 200 elements, 0.6 compared to 0.8 with yours)
pretty stoked to find a use for this niche algorithm, but i wouldn't be surprised if it's possible to do better token wise, perhaps with some add/del tricks like your alg does


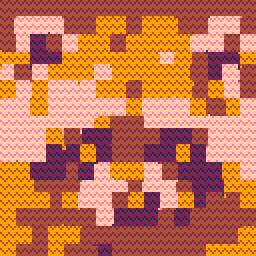
oh, I recognized that sort before I clicked the link! I love it, it's so funny.
the add/deli trick doesn't work as-is unfortunately -- it requires i<=j. that's why I didn't look into using your sort, but clearly I should have -- nice token savings!
[Please log in to post a comment]