A GUI library for Picotron!
It includes basic components: boxes, text boxes, text input, buttons, sliders, radio and multiple selection buttons, and color selecter. And layout components: vertical and horizontal stacks, dropdowns, a topbar and a scrollable container.
It can be useful to test game mechanics or to build app interfaces.
You can use this cart and see how it works or check the documentation on the github repository.
(I'll try to make a video on how to use it and post it here too :))
Here's one simple example:
Let me know if you use it for any projects or if you have improvement ideas!
Note: keyboard text input is not currently working on the online version
Changelog:


That looks great and I'll definitely use it for my game, if I get to a point where UI is needed, which will be a while


@MaddoScientisto Great! Let me know when you use it and if you have any questions or feedback


This is great!
One thing I noticed is there's no way mentioned in the docs to close a dropdown on a button press, so I'm doing something like this, based on source code:
local topbar_options = { {"button",{text="File",stroke=false}}, {"dropdown",{label="top_tools",text="Tools",stroke=false,contents={ {"button",{text="Level Editor",stroke=false}}, {"button",{text="Soundscape Editor",stroke=false}}, }}}, {"button",{text="About",stroke=false}} } local topbar = pgui:component("topbar",{contents=topbar_options}) if topbar[2][1] then pgui:set_store("top_tools",false,true) include "devtools/leveleditor.lua" elseif topbar[2][2] then pgui:set_store("top_tools",false,true) include "devtools/soundscape.lua" end |
It works, but does that look right?
Thanks for the awesome work


hi @x_k, you're absolutely right!
Just so other people understand what is happening:
pgui:set_store("top_tools",false,true) |
Sets to false the internal store that keeps track of opening/closing the dropdown with the "top_tools" label. (The last argument of the function is used to choose between the internal store of pgui or a user's store that can also be used, true is for pgui's store, false for user's store).
Thank you for pointing this out, I might create a function to close or open the dropdown to facilitate the process, but you figured it out perfectly. Maybe I can just explain this method in the docs.


I just started using this to start making my childhood dream: a debug menu
Very easy to implement, I'm glad usage of camera() does not mess up the positioning of the UI interactions, I just have to make sure that I draw the UI after I reset camera and not while it's shifted, but since most of the logic is happening in update this is really easy to do
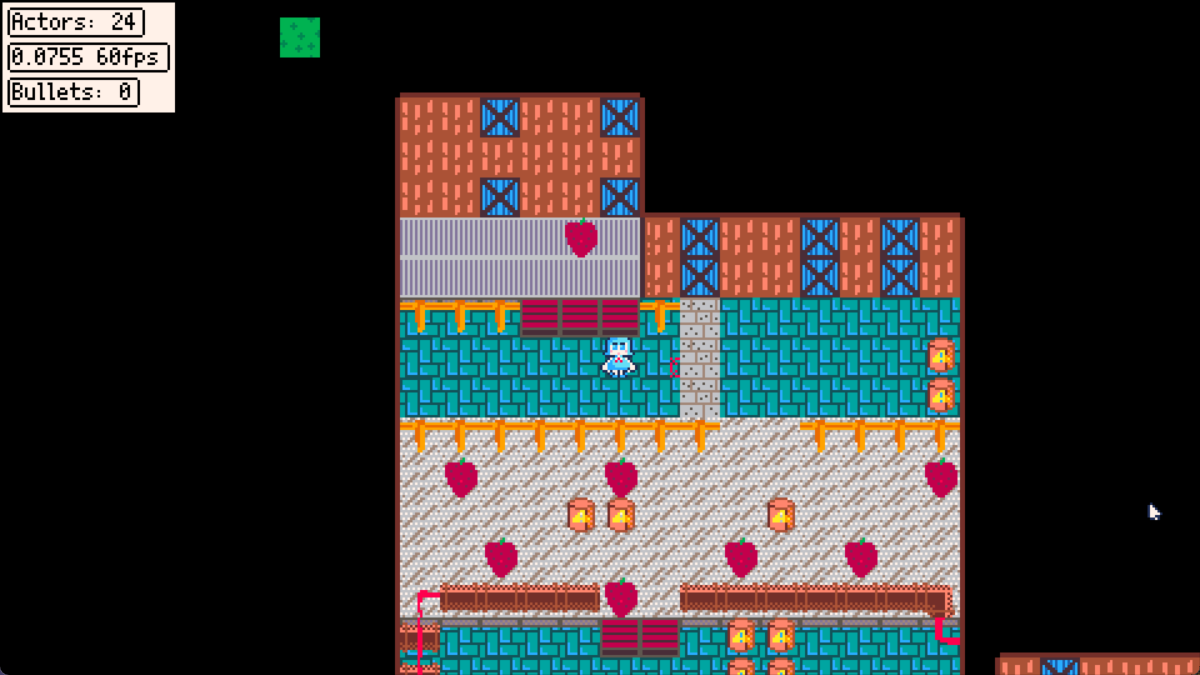


@MaddoScientisto Nice! And I like the design of the character


Everything is pretty much temp sprites, the character was randomly made by a friend of mine.
By the way, I tried to make the background of the GUI transparent by using color= {0,18,12,0,7,6}
but nothing changed, any idea why?
pgui:component("vstack",{stroke=true,pos=vec(0,0),color={0,18,12,0,7,6},height=0,margin=3,gap=3,contents={ {"text_box",{text=string.format("x: %.2f y: %.2f", cirnoInstance.x, cirnoInstance.y),margin=2,stroke=true,active=false,hover=false},color={0,18,12,0,7,6}}, {"text_box",{text=string.format("mx: %.2f my: %.2f", cirnoInstance.move_x, cirnoInstance.move_y),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("Actors: %d", count(actors)),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("%.4f %dfps",stat(1),stat(7)),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("Bullets: %d", count(bullets)),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("Particles: %d", count(particles)),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("Weapon: %s", cirnoInstance.weapon.data.name),margin=2,stroke=true,active=false,hover=false}}, {"text_box",{text=string.format("Weapon timer: %d", cirnoInstance.weapon.timer),margin=2,stroke=true,active=false,hover=false}}, {"sprite_box",{sprite=cirnoInstance.weapon.data.spriteIndex,margin=2,stroke=true,active=false,hover=false}}, }}) |
This is what the debug hud looks like now
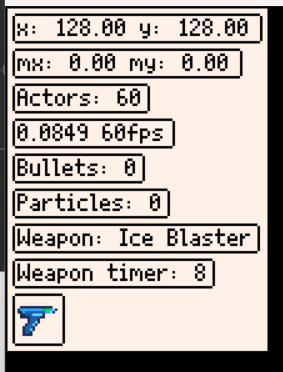


@MaddoScientisto The main reason is that, when you draw shapes, 0 is actually not transparent but black (in the default palette). On the contrary, 0 is transparent on sprites unless you use the palt
function to make the color visible.
However, I did miss to implement the part where color palettes are passed from one component onto its children components, I'll have to add that. In the meantime, you can change the general palette with the function: pgui:set_palette(YOUR_PALETTE_TABLE)
, you can put it inside the _init function.
And if you don't want to draw the background box in the vstack, you can comment out the part of the component that draws it:
It will keep the structure (margins, gaps, etc., but without the box behind).
Btw, if you're happy with the default options for a component (like margins, stroke, etc.), you can omit them when you create it, this can make the code more readable. For instance, in your example:
[Please log in to post a comment]